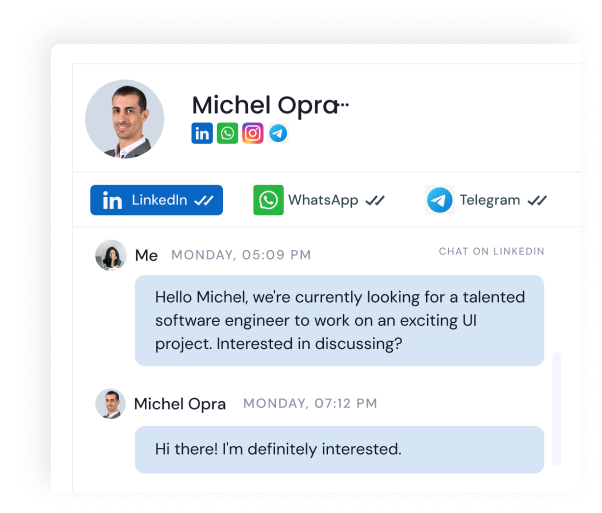
Integrating the LinkedIn API unlocks powerful features for SaaS providers, including CRM, ATS, and outreach software. Whether your goal is to automate prospecting, sync conversations, or enrich user profiles, leveraging this API is a game-changer.
LinkedIn API allows for seamless workflow automation, deeper engagement with LinkedIn’s professional network, and improved efficiency in handling business operations. Learn more about LinkedIn API and its best integration practices.
Why integrate LinkedIn API into your software?
Automated connection management (sending invites, tracking contacts, and removing inactive connections).
Enhanced user engagement with LinkedIn messaging features, including automated follow-ups, response tracking, and sentiment analysis.
Optimized prospecting by syncing interactions with CRM and ATS, improving the accuracy of lead targeting and response rates.
Better campaign performance tracking within LinkedIn, allowing data-driven decision-making for outreach efforts.
Seamless integration with other tools, such as email marketing software and sales automation platforms, creating a unified workflow.
Improved candidate engagement for recruitment software, allowing personalized and automated outreach based on candidate activity.
Understanding LinkedIn API access
Official LinkedIn APIs
- Profile API: Retrieve public user profile data, including skills, experience, and endorsements, which helps personalize user interactions.
- Connections API: Access a user’s network connections, gain insights into shared connections, and establish meaningful relationships.
- Messaging API: Send and receive LinkedIn messages, automate responses, manage message histories, and improve response efficiency.
- UGC API: Manage and publish LinkedIn content, including rich media, sponsored posts, and company updates to enhance visibility.
- Organization API: Handle company pages and job postings, automate job listings, manage company updates, and monitor engagement metrics.
Three ways to access LinkedIn data
1. Become an official LinkedIn partner
This is the most legitimate and stable approach but requires a lengthy approval process, strict compliance policies, and ongoing monitoring to ensure API usage aligns with LinkedIn’s guidelines.
2. DIY (Do It Yourself) approach
Using reverse engineering or automation scripts comes with risks, including account bans, compliance issues, limited scalability, and increased maintenance efforts due to frequent LinkedIn updates.
3. Use a unified API solution
Third-party APIs offer easier integration, unified access, and better compliance by abstracting LinkedIn’s restrictions and handling complex authentication flows. These solutions simplify implementation while ensuring adherence to data security best practices.
Step-by-Step guide to LinkedIn API integration with unipile
1. Set up your unipile developer account
Unipile simplifies LinkedIn API integration by offering a unified API that manages authentication and data synchronization. To get started:
- Sign up on Unipile’s Dashboard.
- Navigate to the API section and create a new application.
- Retrieve your Data Source Name (DSN) and API credentials from the Unipile dashboard.
2. Authenticate and retrieve an access token
Unlike LinkedIn’s official API, which requires a complex OAuth process, Unipile streamlines authentication using a headless browser approach. This allows seamless login and synchronization without needing LinkedIn’s official partner approval.
Here’s how to generate an access token using Unipile’s API:
const axios = require('axios');
const getAccessToken = async () => {
const response = await axios.post('https://api.unipile.com/auth/token', {client_id: 'your-client-id',
client_secret: 'your-client-secret'
});
return response.data.access_token;
};
This access token grants secure and compliant access to LinkedIn messaging, profile data, and other API features.
3. Fetch LinkedIn data using Unipile API
Once authenticated, you can retrieve LinkedIn profile information, messages, and chats directly through Unipile’s API. Instead of handling LinkedIn’s rate limits and authentication complexities, Unipile manages everything in the background.
Example: Fetching a LinkedIn Profile
const getProfile = async (accessToken, profileId) => {
const response = await axios.get(`https://api.unipile.com/linkedin/profile/${profileId}`, {
headers: { 'Authorization': `Bearer ${accessToken}` }
});
return response.data;
};
With Unipile, you do not need to apply for LinkedIn’s restricted API access. The platform automates profile data retrieval and messaging features.
4. Sync LinkedIn conversations in real-time
Unipile offers webhooks to automatically sync LinkedIn messages and contacts with your CRM, ATS, or outreach software.
Example: Listening for new LinkedIn messages
const listenForMessages = async () => {
const response = await axios.get('https://api.unipile.com/linkedin/messages', {
headers: { 'Authorization': `Bearer ${accessToken}` }
});
console.log(response.data);
};
This allows real-time updates on user conversations without manual fetching.
5. Automate LinkedIn outreach with Unipile
Unipile supports automated messaging, connection requests, and follow-ups, ensuring compliance with LinkedIn’s guidelines while optimizing engagement.
Example: Sending an automated LinkedIn message
const sendMessage = async (accessToken, recipientId, message) => {
const response = await axios.post('https://api.unipile.com/linkedin/message', {
recipient_id: recipientId,
content: message
}, {
headers: { 'Authorization': `Bearer ${accessToken}` }
});
return response.data;
};
This is useful for recruitment automation, lead nurturing, and follow-up sequences within outreach campaigns.
Advanced LinkedIn API features
Webhooks for real-time data synchronization and proactive engagement tracking, improving automation workflows.
AI-driven insights for lead scoring, helping prioritize high-value connections based on user activity and interactions.
Integration with multi-channel platforms like WhatsApp, email, and Slack for a seamless user experience across different communication methods.
Automated data enrichment by pulling LinkedIn insights into CRM platforms, improving lead profiling and personalization.
Bulk messaging capabilities with rate-limiting adherence to avoid account restrictions and maintain compliance.
Advanced analytics on LinkedIn activity, helping businesses monitor campaign performance and optimize messaging strategies.
Security & Compliance considerations
To ensure secure API access and prevent unauthorized use, always authenticate with OAuth 2.0. Protect sensitive data by encrypting API keys and access tokens, reducing the risk of security breaches.
For GDPR compliance, give users control over their data by allowing deletion requests and ensuring explicit consent for data usage. Regularly monitor API logs to spot unusual activity early and avoid account suspension.
Enhance security by whitelisting IP addresses, allowing access only from trusted sources. Finally, audit API permissions regularly to ensure they align with your business needs and follow best security practices.
Common challenges & Solutions
Challenge
Solution
Access restrictions
Use third-party API solutions that handle authentication complexities and ensure compliance with LinkedIn policies.
Rate limits
Optimize API calls by batching requests, caching responses, and using background processing to improve efficiency.
OAuth complexity
Automate token refresh mechanisms and implement secure storage for credentials to prevent authentication failures.
Integration issues
Test API responses with mock data before deploying in production to ensure seamless functionality and avoid disruptions.
User data privacy
Implement strict role-based access controls to restrict sensitive data exposure and comply with data protection regulations.
Scalability concerns
Leverage API load balancing techniques and distributed architecture to handle large volumes of LinkedIn API requests efficiently.
Final thoughts
Integrating LinkedIn API is powerful but challenging. While becoming a LinkedIn partner or building a DIY solution is complex, using a third-party API simplifies access while ensuring security, flexibility, and compliance. Proper implementation allows businesses to streamline workflows, improve prospecting, and enhance engagement through LinkedIn’s vast professional network. By leveraging advanced features, maintaining security best practices, and optimizing API usage, businesses can unlock the full potential of LinkedIn integration for sales, recruiting, and marketing automation.
Leave a Reply